This tutorial is focused on Create Restful CRUD API using Node js Express MongoDB , Later on we will use these api method in our Frontend Application in Angular 6.
So lets learn how to create restful service in nodejs with express framework, and Perform Crud operations in MongoDBdata.
Prerequisites for this Application:
1) Node and Npm should be Installed in system. Following is the download Download link/instructions
https://nodejs.org/en/
2) Mongo Db should be Installed in the system. Following is the download link/instructions for it
https://www.mongodb.com/
Step 1) Create directory and Initialize NPM
mkdir my_node_app cd my_customers npm init
After ‘Npm Init’ run it will ask for some meta information, Some fields (like test command) are optional you may leave blank by entering without any text.
In main script you need to provide the main bootstrap file for your application , i will provide `index.js` as main file name here.
After providing the required information it will have a composer.json file in your project like following
{ "name": "mycustomers", "version": "1.0.0", "description": "manage my customers", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "keywords": [ "manage", "clients" ], "author": "jeetendra", "license": "ISC" }
Step 2) Following dependencies we need to install in our project:
- Express framework
- Mongoose module to communicate with our already installed mongo server
- Body-parser module to recieve the form data send by client applications to our api methods
Below are the commands to install above modules
npm install express --save npm install mongoose --save npm install body-parser --save
Step 3) Now create a index.js file to create apis in it
Now create index.js file to create API using Node js Express MongoDB
Load modules and initialize express app following code will load the modules in express app
var express = require('express'); var mongoose = require('mongoose'); var body_parser = require('body-parser'); var app = express();
After it define the database credentials using host and db name and pass that mongodb connection url in mongoose.connect method:
var db_host='localhost' var db_name='customers_db' mongoose.connect('mongodb://'+db_host+'/'+db_name);
After it add following 2 lines to support application/json and application/x-www-form-urlencoded type of post data in apis
// support parsing of application/json type post data app.use(body_parser.json()); //support parsing of application/x-www-form-urlencoded post data app.use(body_parser.urlencoded({ extended: true }));
Step 4) Before moving further we will require to create model for our customers data
So create a folder models and create Customers.js with following code in that file (models/Customers.js) :
var mongoose = require('mongoose'); //code will come here to insert customer in mongo database var Schema = mongoose.Schema; // create a schema var CustomerSchema = new Schema({ name: { type: String, required: true }, phone: { type: String, required: true }, email: { type: String, required: true }, age: { type: String, required: true } }); //register as model and export it with name Customers module.exports = mongoose.model('Customers', CustomerSchema)
Step 5) Now in our Index.js File where we already created mongo connection there , after it we put the body parser configuration.
So after that we will include the Model which we created and create the required apis for crud oprations
var Cutomer_Model = require('./models/Customers');
After it Assign a port to your application for handeling client requests using following code
var server = app.listen(8080, function () { console.log("Application Server Started listening requests"); });
Now we will create API using Node js Express MongoDB for Create,Read,Update,Delete Operations that will use in Angular js 6 later on
Api 1: Create Customers using POST Request:
Endpoint Name : create_customer
app.post('/create_customer', function (req, res) { var user_obj = new Cutomer_Model(req.body); var return_arr={}; user_obj.save(function(err) { if (err) { return_arr.status=0; return_arr.message=err.message; } else { return_arr.status=1; return_arr.message="Customer Created Successfully"; } var ReturnjsonString = JSON.stringify(return_arr); res.json(ReturnjsonString); }); });
in above method we defined the app post method with create_customer is a endpoint name.
where we will post the data as we defined the Schema for mongo db . after it we will receive that posted data using
req.body. Now create a object for Customer model by passing the request body to its Customers Model class constructer. It will returning a customer object and using that object we are calling save method and returning the response accordingly in callback method
Api 2: Select all customers using GET Request
Endpoint Name : get_all_customers
app.get('/get_all_customers', function (req, res) { //select all records, just intantiate the modal with its name Cutomer_Model.find({}, function(err, data) { var return_arr={}; if (err) { return_arr.status=0; return_arr.message=err.message; } else { return_arr.status=1; return_arr.customers=data; } res.json(return_arr); }); });
Api 3: Update customer using PUT Request
Endpoint Name : update_customer
app.put('/update_customer/:customer_id', function (req, res) { var return_arr={}; Cutomer_Model.findByIdAndUpdate(req.params.customer_id,req.body,{new: true},function(err) { if (err) { return_arr.status=0; return_arr.message=err.message; } else { return_arr.status=1; return_arr.message="Customer Updated Successfully"; } res.json(return_arr); }); });
Api 4: Delete customer using Delete Request
Endpoint Name : delete_customer
app.delete('/delete_customer/:customer_id', function (req, res) { var return_arr={}; Cutomer_Model.findByIdAndRemove(req.params.customer_id,function(err) { if (err) { return_arr.status=0; return_arr.message=err.message; } else { return_arr.status=1; return_arr.message="Customer Deleted Successfully"; } res.json(return_arr); }); });
Thanks to reading out this article focuses on Creating Resful API using Node js Express MongoDB
Apart from above scenarios there may be multiple requirements, like search with name etc fields or get specific data with id. If you need any type of help or something not clear to you, then you may put your comments below.
Click here to download full source code for create API using Node js Express MongoDB
You are now ready to with API using Node js Express MongoDB .
Following are the Postman Screenshots to test these apis respectively
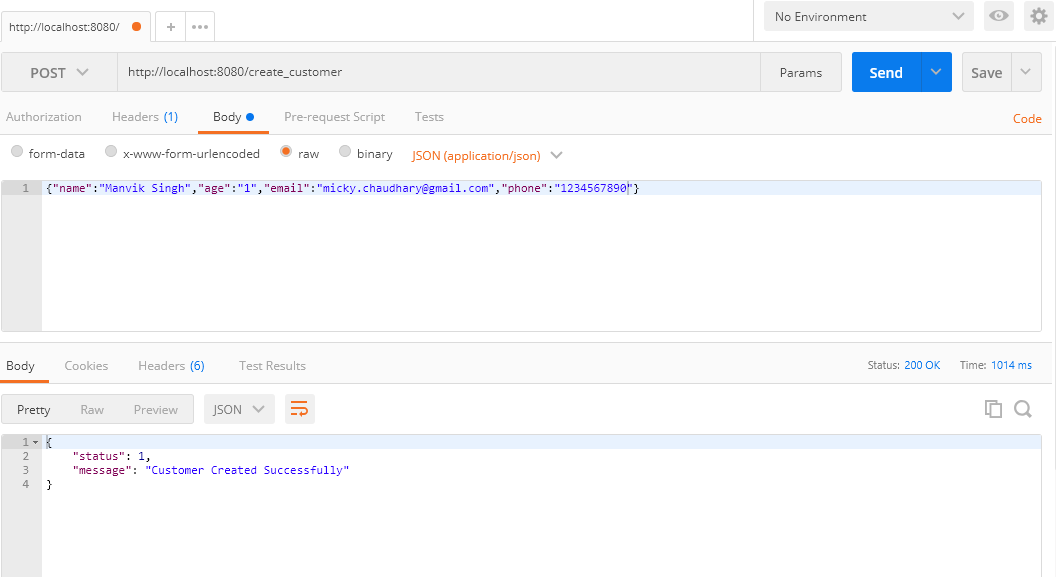
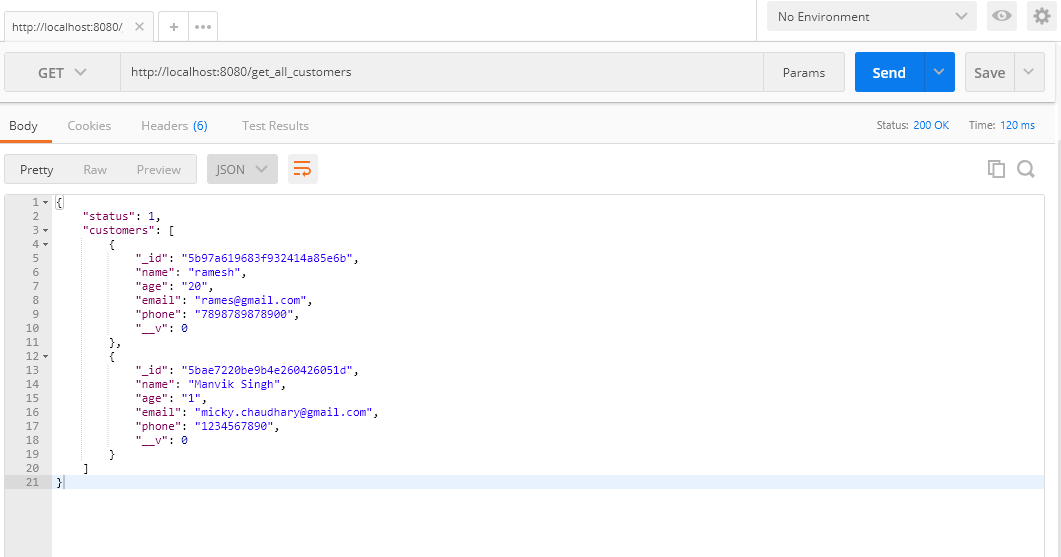
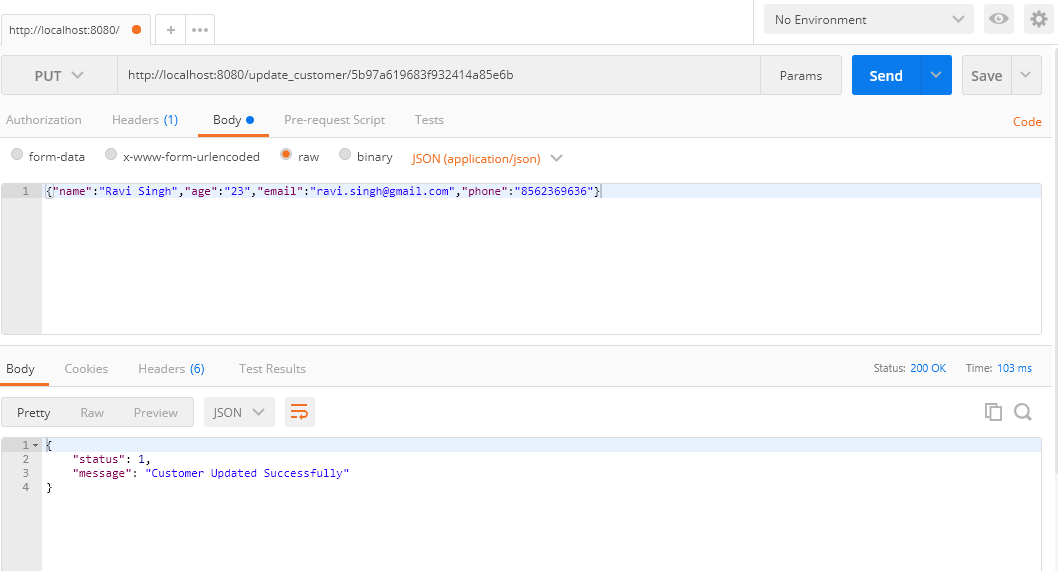
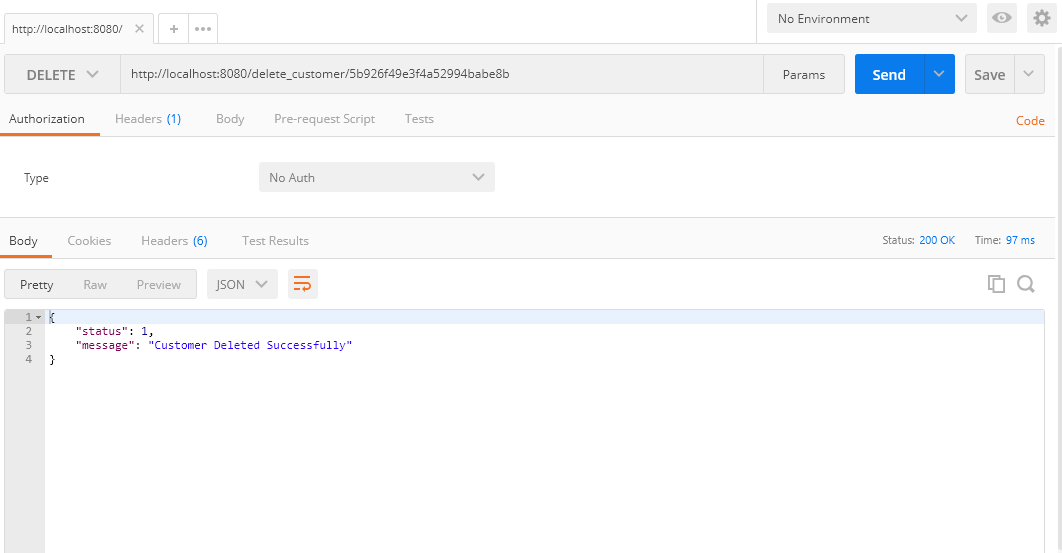