Hi Geeks,
I am Sharing you the code for reading a rss feed using php script.
Following are the Steps to make a news aggregator website using rss reader in php
Step 1: Create a form having Rss Urls in select box as following
<p><b>Choose category :</b> <form method="post" id="myform" > <select required name="rssurl"> <option value="">Select</option> <option value="http://timesofindia.feedsportal.com/c/33039/f/533916/index.rss">India News</option> <option value="http://timesofindia.feedsportal.com/c/33039/f/533917/index.rss">World News</option> <option value="http://timesofindia.feedsportal.com/c/33039/f/533922/index.rss">Science News</option> </select> <input type="submit" value="Load" /> </form> </p>
Step 2. Php code to recieve the submitted post and rss url. and get the rss output in object and parse the feed or rss using the dom document class and accessing the properties as following:
<?php if(isset($_POST['rssurl'])) { echo '<h1>Search Result for rss url:'.$_POST['rssurl'].'</h1>'; $rssurl=$_POST['rssurl']; $rss = new DOMDocument(); $rss->load($rssurl); $feed = array(); foreach ($rss->getElementsByTagName('item') as $node) { $item = array ( 'title' => $node->getElementsByTagName('title')->item(0)->nodeValue, 'desc' => $node->getElementsByTagName('description')->item(0)->nodeValue, 'link' => $node->getElementsByTagName('link')->item(0)->nodeValue, 'date' => $node->getElementsByTagName('pubDate')->item(0)->nodeValue, ); array_push($feed, $item); } $limit = 5; for($x=0;$x<$limit;$x++) { $title = str_replace(' & ', ' & ', $feed[$x]['title']); $link = $feed[$x]['link']; $description = $feed[$x]['desc']; $date = date('l F d, Y', strtotime($feed[$x]['date'])); echo '<p><strong><a href="'.$link.'" title="'.$title.'">'.$title.'</a></strong><br />'; echo '<small><em>Posted on '.$date.'</em></small></p>'; echo '<p>'.$description.'</p>'; } } ?>
Final Complete Code:
<p><b>Choose category :</b> <form method="post" id="myform" > <select required name="rssurl"> <option value="">Select</option> <option value="http://timesofindia.feedsportal.com/c/33039/f/533916/index.rss">India News</option> <option value="http://timesofindia.feedsportal.com/c/33039/f/533917/index.rss">World News</option> <option value="http://timesofindia.feedsportal.com/c/33039/f/533922/index.rss">Science News</option> </select> <input type="submit" value="Load" /> </form> </p> <?php if(isset($_POST['rssurl'])) { echo '<h1>Search Result for rss url:'.$_POST['rssurl'].'</h1>'; $rssurl=$_POST['rssurl']; $rss = new DOMDocument(); $rss->load($rssurl); $feed = array(); foreach ($rss->getElementsByTagName('item') as $node) { $item = array ( 'title' => $node->getElementsByTagName('title')->item(0)->nodeValue, 'desc' => $node->getElementsByTagName('description')->item(0)->nodeValue, 'link' => $node->getElementsByTagName('link')->item(0)->nodeValue, 'date' => $node->getElementsByTagName('pubDate')->item(0)->nodeValue, ); array_push($feed, $item); } $limit = 5; for($x=0;$x<$limit;$x++) { $title = str_replace(' & ', ' & ', $feed[$x]['title']); $link = $feed[$x]['link']; $description = $feed[$x]['desc']; $date = date('l F d, Y', strtotime($feed[$x]['date'])); echo '<p><strong><a href="'.$link.'" title="'.$title.'">'.$title.'</a></strong><br />'; echo '<small><em>Posted on '.$date.'</em></small></p>'; echo '<p>'.$description.'</p>'; } } ?>
Conclusion : Select your category and click on go button and you will see the fetched news from rss feed.
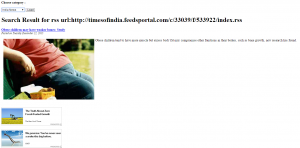